Extraordinary Tech Partnership That Makes a Difference
We are an IT consulting and service provider. With a proven track record of successful projects across various industries, Pionero offers reliable and high-quality solution, leveraging our partners’ expertise and innovation.
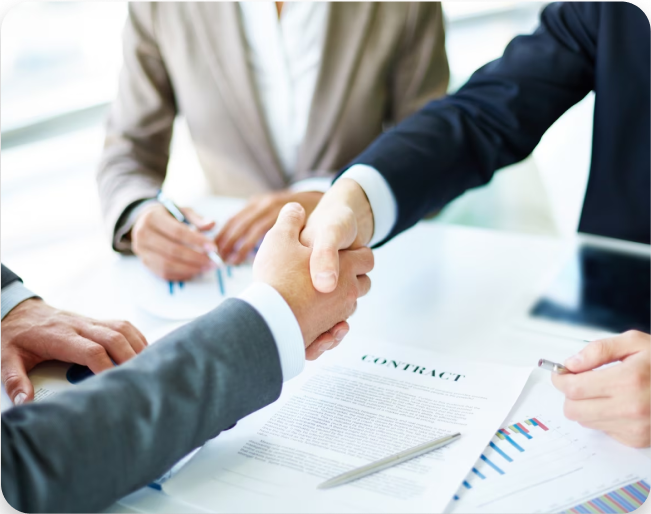
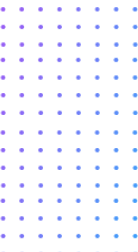
The home of innovation and successs
Embrace Technology for a Happier World at Pionero
At Pionero, we believe in the power of technology to improve lives and create a happier, more fulfilling world. We strive to leverage technological advancements to enhance well-being and foster a more joyful society. This includes using technology to improve communication, education, healthcare, entertainment, and sustainable development. By connecting people, making education and healthcare more accessible, providing enjoyable experiences, and addressing environmental challenges, technology can contribute to greater happiness and a higher quality of life. Our goal is to harness technology’s potential in ethical and responsible ways, ensuring its benefits are accessible to all. We aim to promote a world where technology is a force for positivity and happiness, and where its potential is used to create a brighter future for everyone.
Become a pioneering DX company
At our company, we envision a future where digital transformation is used to enhance well-being and foster happiness on a global scale. We strive to be at the forefront of this transformation, utilizing innovative technologies and practices to revolutionize industries, processes, and experiences. We believe that by leveraging technology as a driving force for positive change, we can create a meaningful and lasting impact on the world. Our commitment to digital transformation is a testament to our belief in the power of technology to connect people, improve lives, and promote sustainability. We aim to lead by example, inspiring others to join us in our mission to create a brighter, happier future for all.
Our Values: Growth, Excellence, and Impact
We value a challenging spirit and are committed to continuous growth, both as individuals and as a company. We strive for excellence in all that we do, upholding high standards of professionalism and expertise in our respective fields. We also believe in the power of technology to create meaningful impact and are dedicated to leveraging it to positively transform the world. These values guide our actions and decision-making, inspiring us to continuously improve, innovate, and make a difference in the lives of those we serve.
What Pionero can do
We cover everything from technology selection proposals to system construction and human resources education and training.We provide digital technology support and support to reduce the burden on companies.
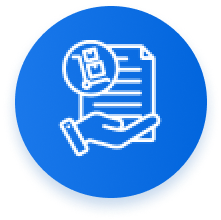
Strategic IT Consulting
Merge ideas and technology into concrete strategy
We seek the appropriate answers, discuss possibilities, and devise a strategy. Our solution proposal are tailored to your needs and wants.
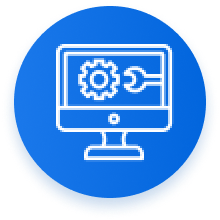
IT Services
Realize the perfect plan with expertise and passion
As technology geeks, we deliver project with openness, speed, and purpose, and we always strive to develop accessible and impactful solutions.
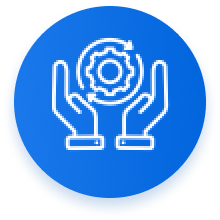
AI Solution R&D
Bring out the best of the latest technology
We harness Artificial Intelligence, Machine Learning, and Natural Language Processing to boost your business performance, increase the efficiency and discover new opportunities.
Welcome to our menu of services
Our company offers a comprehensive range of technology services to help businesses and organizations succeed in the digital age. Our offerings include strategic IT consulting, IT services, and AI R&D solutions, providing expert guidance and support for businesses to optimize their technology infrastructure and leverage cutting-edge innovations.
The following services are offered:
- Strategic IT Consulting
- IT Services
- AI R&D Solutions
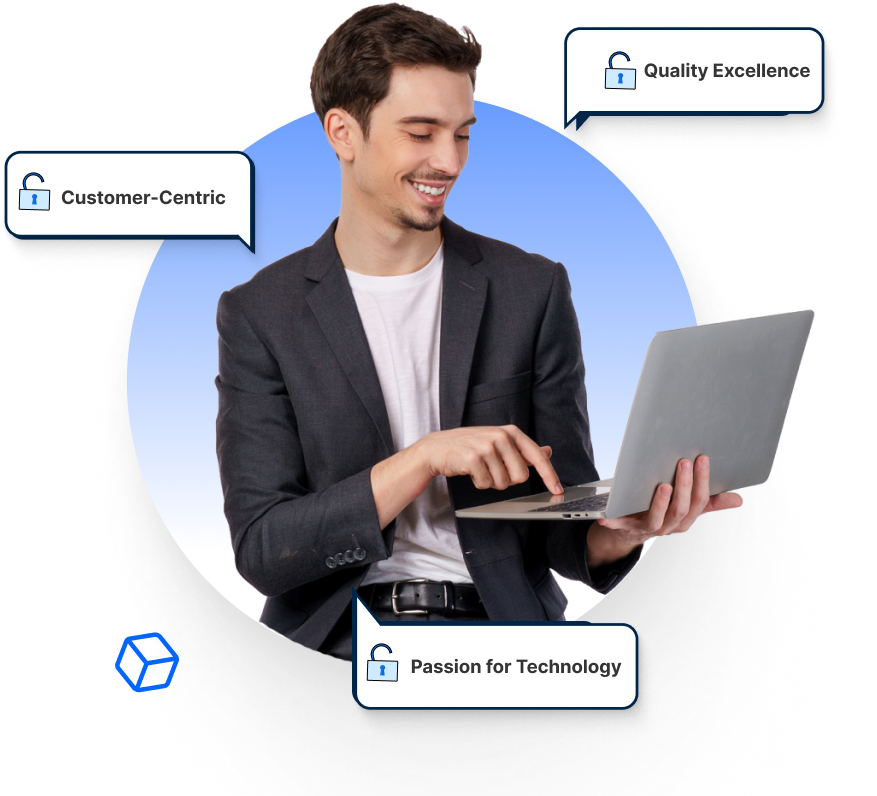
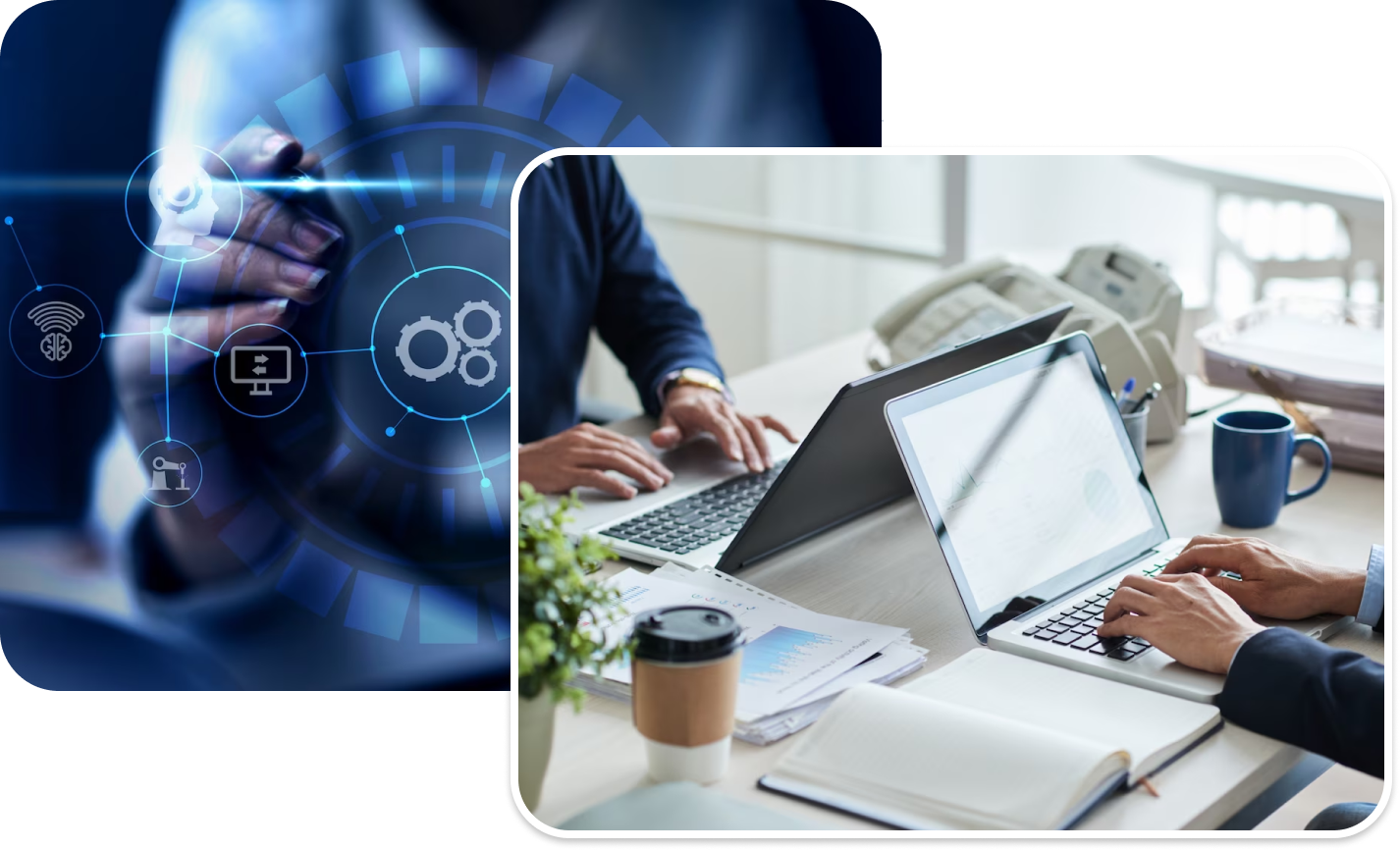
Experience matters
We are experts in finding professional services talent for grawling industries
- Finance
- Retail
- Healthcare
- Education
- Energy and Utilities
- Media and Entertainment
Customer-Centric:
We are committed to understanding and meeting the unique needs of our customers, delivering the best value for their investment.
Quality Excellence:
We uphold the highest standards of performance, reliability, and security, ensuring that our development services exceed expectations.
Passion for Technology:
We are passionate about technology and its potential to drive business success, bringing innovation and enthusiasm to every project we undertake.
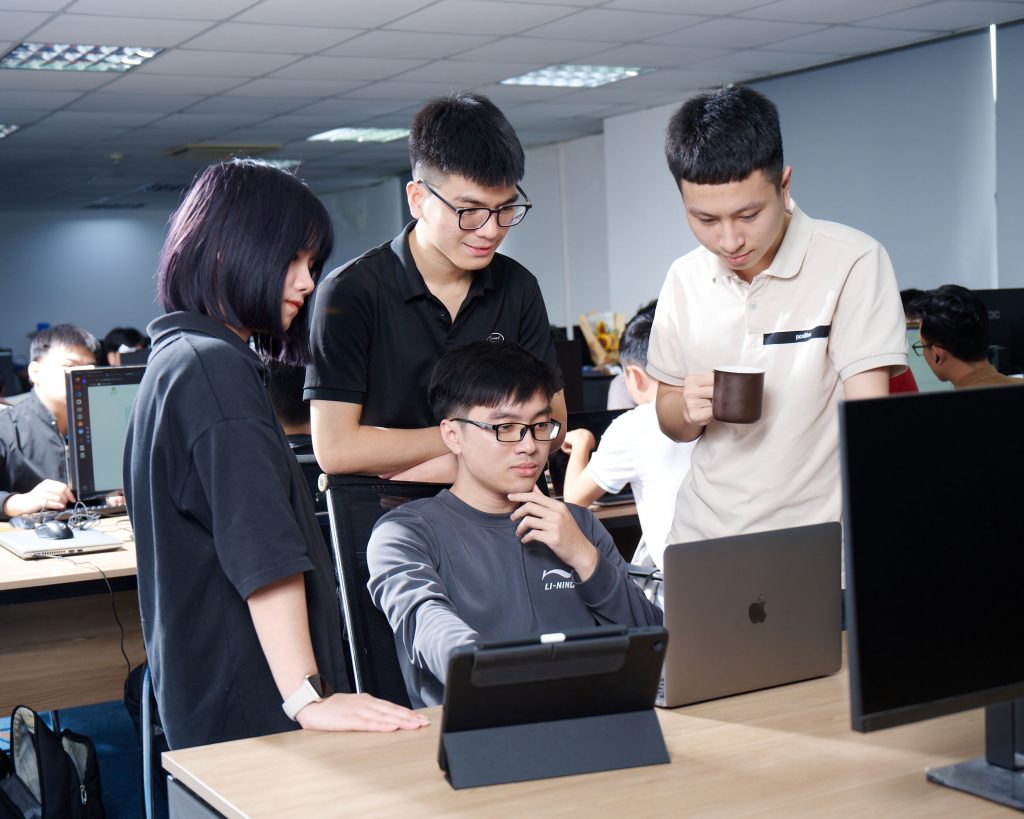
Helping businesses around the world
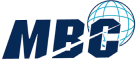
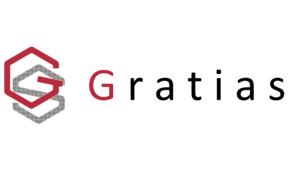
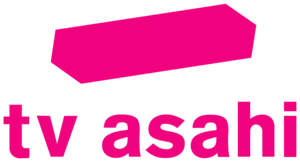
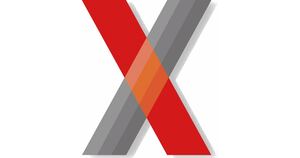
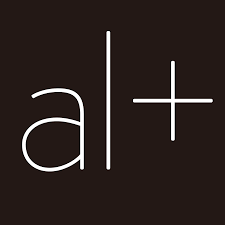
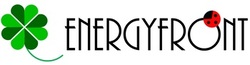
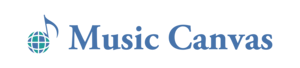
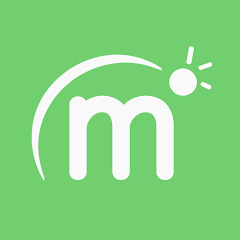
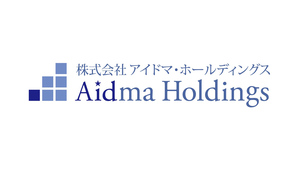
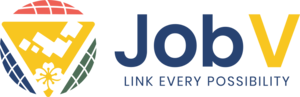



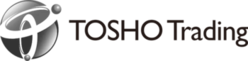

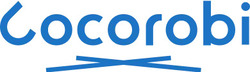
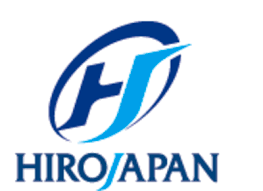
Lastest from our blog
- 03/05/2024
- 17/04/2024
- 04/04/2024
- 04/04/2024
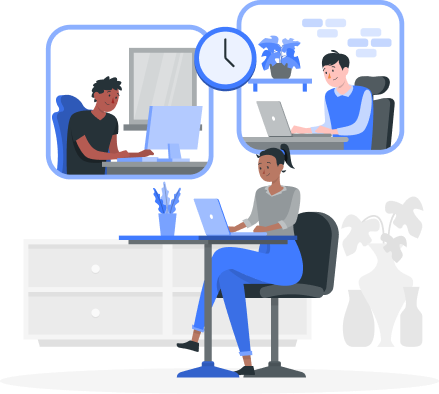